Hello Axum
What is Axum?
- Axum은 Rust를 사용하여 서버측 웹 애플리케이션을 구축하기 위한 프레임워크입니다 .
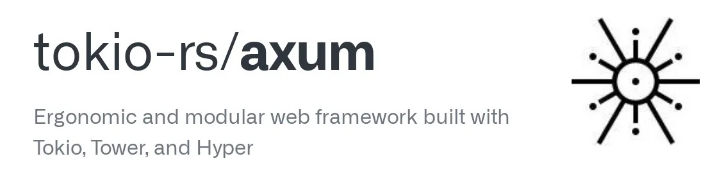
설치
- Axum프로젝트를 위해서 먼저 Rust프로젝트를 만들어줍니다.
- rust가 설치되어 있지 않다면 Rust를 먼저 설치해줍니다.
Rust install
1
2
3
| [dependencies]
tokio = { version = "1.36.0", features = ["full"] }
axum = "0.7.5"
|
Hello Axum
- 디렉토리에는 main.rs와 lib.rs가 있어야합니다.

1
2
3
4
5
6
7
8
9
| //main.rs
use axum_login::run;
#[tokio::main]
async fn main(){
run().await;
}
|
- lib.rs에 run 함수를 작성해줍니다.
- Axum에 Router을 작성하며 “/”는 기본 페이지입니다.
- axum::serve()를 통해 127.0.0.1:3000에 서버를 시작합니다.
1
2
3
4
5
6
7
8
9
10
| //lib.rs
pub async fn run() {
let app = Router::new().route("/", get(index));
let listener = tokio::net::TcpListener::bind("127.0.0.1:3000")
.await
.unwrap();
println!("listening on {}", listener.local_addr().unwrap());
axum::serve(listener, app).await.unwrap();
}
|
- index.html을 작성해줍니다.메인페이지가 될것입니다.
1
2
3
4
5
6
7
8
9
10
11
| <!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
|
을 입력하게 되면
1
| listening on 127.0.0.1:3000
|
이 뜨게 되며 “http://localhost:3000/” 에 접속하게 되면 Hello world가 맞이할것입니다.
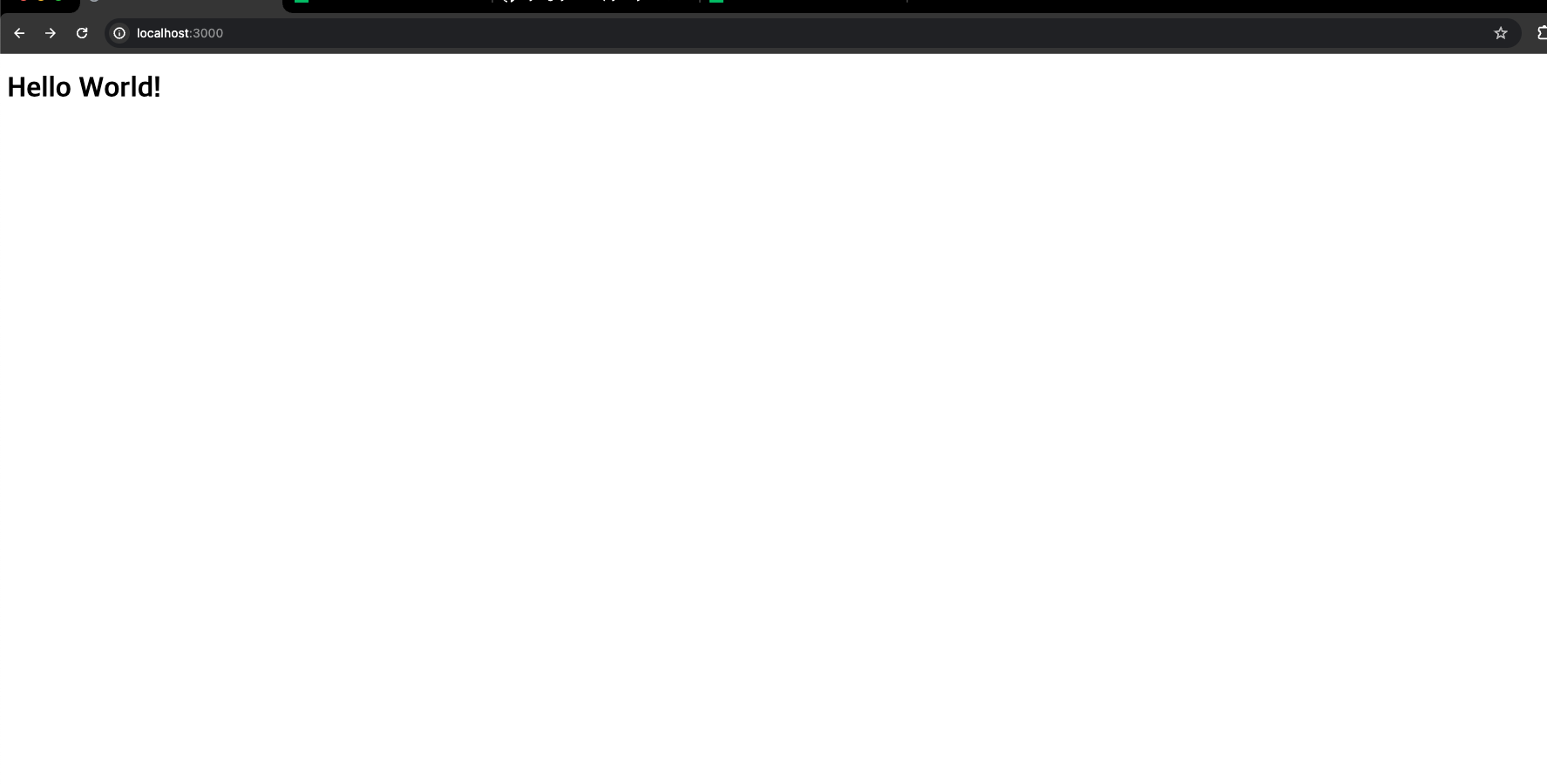
전체코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
pub mod db;
pub mod model;
pub mod router;
use axum::{response::Html, routing::get, Router};
use tokio;
pub async fn run() {
let app = Router::new().route("/", get(index));
let listener = tokio::net::TcpListener::bind("127.0.0.1:3000")
.await
.unwrap();
println!("listening on {}", listener.local_addr().unwrap());
axum::serve(listener, app).await.unwrap();
}
async fn index() -> Html<&'static str> {
let html_content = include_str!("../index.html");
Html(html_content)
}
|